|
发表于 2022-11-26 19:51:17
|
显示全部楼层
1.Math和Mathf:
概念:
- Math是C#中封装好的用于数学计算的工具类 —— 位于System命名空间中
- Mathf是Unity中封装好的用于数学计算的工具结构体 —— 位于UnityEngine命名空间中
- 它们都是提供来用于进行数学相关计算的
区别:
- Mathf 和 Math中的相关方法几乎一样
- Math是C#自带的工具类 主要就提供一些数学相关计算方法
- Mathf是Unity专门封装的,不仅包含Math中的方法,还多了一些适用于游戏开发的方法
- 所以我们在进行Unity游戏开发时
- 使用Mathf中的方法用于数学计算即可
2.Mathf中的常用方法—— 一般计算一次:
Debug.Log(Mathf.PI);
//取绝对值
Debug.Log(Mathf.Abs(-10));
//向上取整
Debug.Log(Mathf.CeilToInt(1.5f));
//向下取整
Debug.Log(Mathf.FloorToInt(1.5f));
//钳制函数
//传入参数 最小值 最大值
//如果传入参数小于最小值,则返回最小值
//如果传入参数大于最大值,则返回最大值
//在范围内则返回传入参数本身
Debug.Log(Mathf.Clamp(10, 5, 20));
//最大值
Debug.Log(Mathf.Max(2, 6, 8));
//最小值
Debug.Log(Mathf.Min(2, 6, 8));
//一个数的n次幂
//数 幂数
Debug.Log(Mathf.Pow(2, 4));
//四舍五入
Debug.Log(Mathf.RoundToInt(1.5f));
//平方根
Debug.Log(Mathf.Sqrt(4));
//判断一个数是否是2的n次方
Debug.Log(Mathf.IsPowerOfTwo(4));
//判断正负数
//正数返回1
//负数返回-1
Debug.Log(Mathf.Sign(1.5f));
Debug.Log(Mathf.Sign(-1));
3.Mathf中的常用方法 —— 一般不停计算:
//插值计算 Lerp
//Lerp函数公式
//result = Mathf.Lerp(start,end,t);
//t为插值系数 取值范围为0 - 1
//result = start + (end - start) * t
//插值运算用法一
//每帧改变start的值——变化速度先快后慢 位置无限接近 但是不会得到end位置
//此时t是不变的,start在不停变化
start = Mathf.Lerp(start, 10, Time.deltaTime);
//插值运算用法二
//每帧改变t的值——变化速度匀速,位置每帧接近,但当t>=1时,得到结果
//此时start不变,time在不停变化
time += Time.deltaTime;
result = Mathf.Lerp(start, 10, time);
4.角度和弧度:
概念:
角度和弧度都是度量角的单位(弧度 > 角度)。
- 角度:1°
- 弧度:1 radian
- 圆一周的角度:360°
- 圆一周的弧度:2π radian
关系:
- π rad = 180°
- 1 rad = (180/π)° ≈ 57.3°
- 1° = (π/180) rad ≈ 0.01745 rad
由此可得出:
- 弧度 * 57.3 = 对应角度
- 角度 * 0.01745= 对应弧度
5.三角函数:
- 三角函数是初等函数之一。
- 包括正弦函数(sin)、余弦函数(cos)、正切函数(tan)等(弧度求sin/cos)。
sin函数:
sin角 = 角的对边 / 角的斜边
cos函数:
sin角 = 角的邻边 / 角的斜边
sin和cos函数曲线:
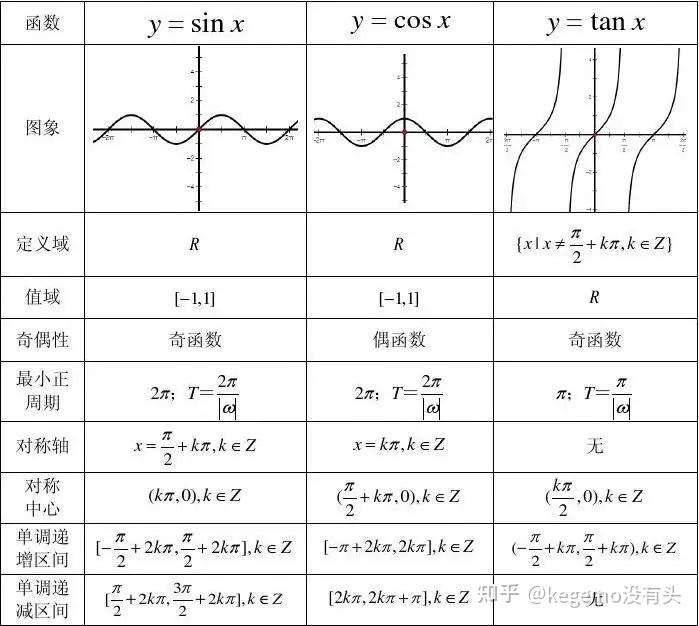
三角函数曲线和相关参数
语法:
//注意:Mathf中的三角函数相关函数 传入的参数需要是弧度值
Debug.Log(Mathf.Sin(rad));
Debug.Log(Mathf.Cos(angle * Mathf.Deg2Rad));
反三角函数:
- 包括反正弦函数、反余弦函数等。
- 通过反三角函数计算正弦值和余弦值对应的弧度值(sin/cos值转弧度)。
float sinRad = Mathf.Sin(rad);
float tempRad = Mathf.Asin(sinRad);
Debug.Log(tempRad);
Debug.Log(tempRad * Mathf.Rad2Deg);
5.坐标系:
世界坐标系:
Debug.Log(transform.position);
Debug.Log(transform.eulerAngles);
Debug.Log(transform.lossyScale);
Debug.Log(transform.rotation);
物体坐标系:
- 原点:物体的中心点
- 轴向:物体右方是x轴正方向、物体上方是y轴正方向、物体前方是z轴正方向
Debug.Log(transform.localPosition);
Debug.Log(transform.localEulerAngles);
Debug.Log(transform.localRotation);
Debug.Log(transform.localScale);
屏幕坐标系:
- 原点:屏幕左下角
- 轴向:向右为x轴正方向、向左为y轴正方向
- 最大宽高:Screen.width;Screen.height;
Debug.Log(Input.mousePosition);
Debug.Log(Screen.width);
Debug.Log(Screen.height);
视口坐标系:
- 原点:屏幕左下角
- 轴向:向右为x轴正方向、向左为y轴正方向
- 特点:左下角为(0,0),右上角为(1,1),和屏幕坐标类似,将坐标单位化
坐标系转换:
//世界转本地
Debug.Log(transform.InverseTransformPoint(transform.position));
//本地转世界
Debug.Log(transform.TransformPoint(transform.localPosition));
//世界转屏幕
Camera.main.WorldToScreenPoint(transform.position);
//屏幕转世界
Camera.main.ScreenToWorldPoint(Camera.main.WorldToScreenPoint(transform.position));
//世界转窗口
Camera.main.WorldToViewportPoint(transform.position);
//视口转屏幕
Camera.main.ScreenToViewportPoint(Camera.main.ScreenToWorldPoint(Camera.main.WorldToScreenPoint(transform.position)));
//屏幕转视口
Camera.main.ViewportToScreenPoint(Camera.main.ScreenToViewportPoint(Camera.main.ScreenToWorldPoint(Camera.main.WorldToScreenPoint(transform.position)))); |
|